We added a new section in the Next.js Docs: Caching
Length: • 2 mins
Annotated by duzhi tan
We added a new section in the Next.js Docs: Caching
↳ nextjs.org/docs/app/build...
Here's the TLDR for those who want a better understanding of the different caching layers and how they relate to Server Components and newer APIs:

You can think of caching like an onion, where each layer reduces network requests to the next layer.
Next.js aims to provide sensible caching defaults and minimal configuration for the best user experience, performance, and cost.
📦 Router Cache
On the client, Next.js stores the RSC Payload (split by route segment) of visited and prefetched routes in a temporary, client-side cache.
This allows snappy navigation, preserves state, and avoids asking the server for UI we've already rendered.
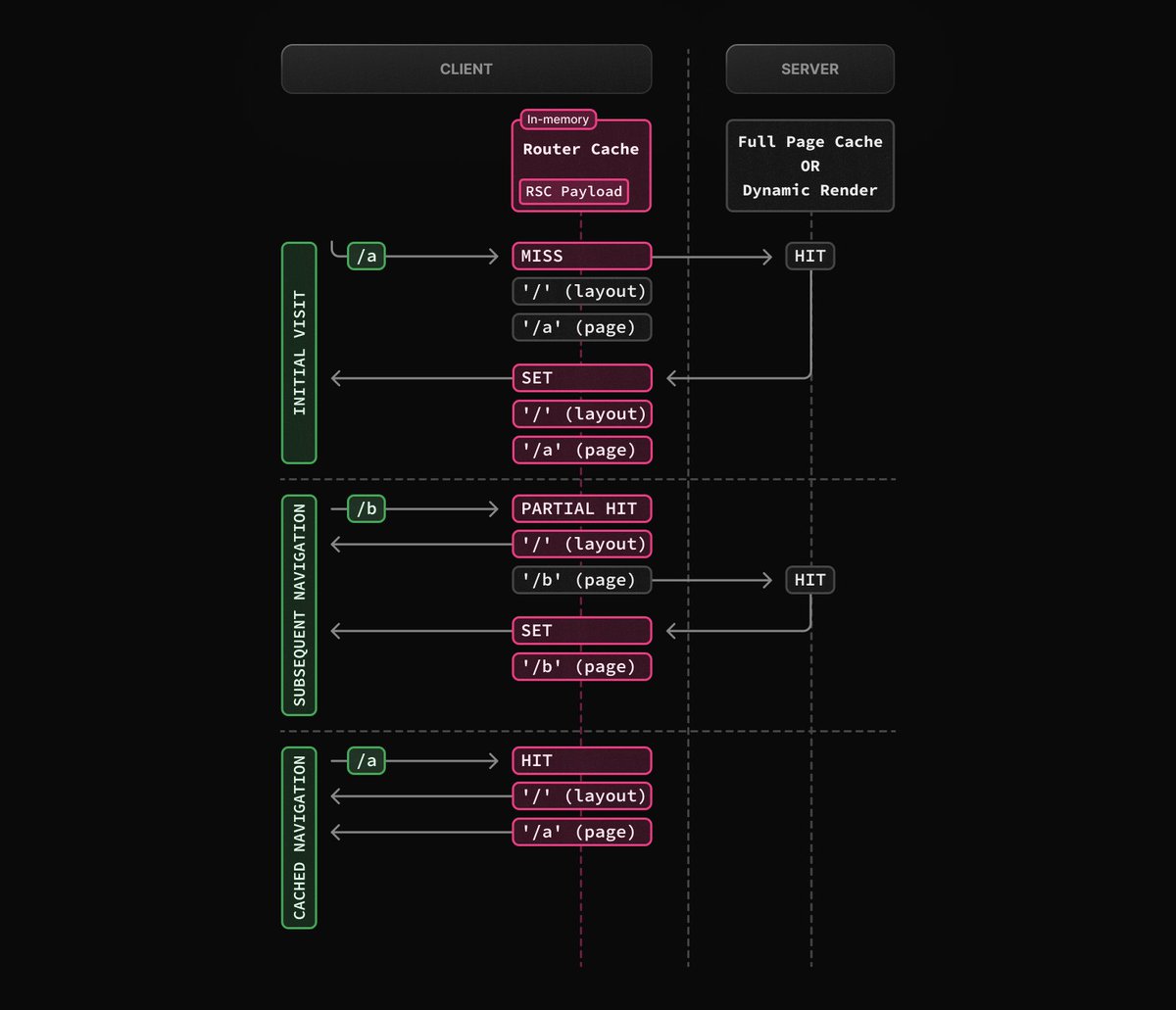
📦 Full Route Cache
If a route doesn't rely on request-time information such as request headers or user cookies, Next.js automatically moves the rendering work to build-time and caches the result in a geographically distributed, persistent server-side cache.
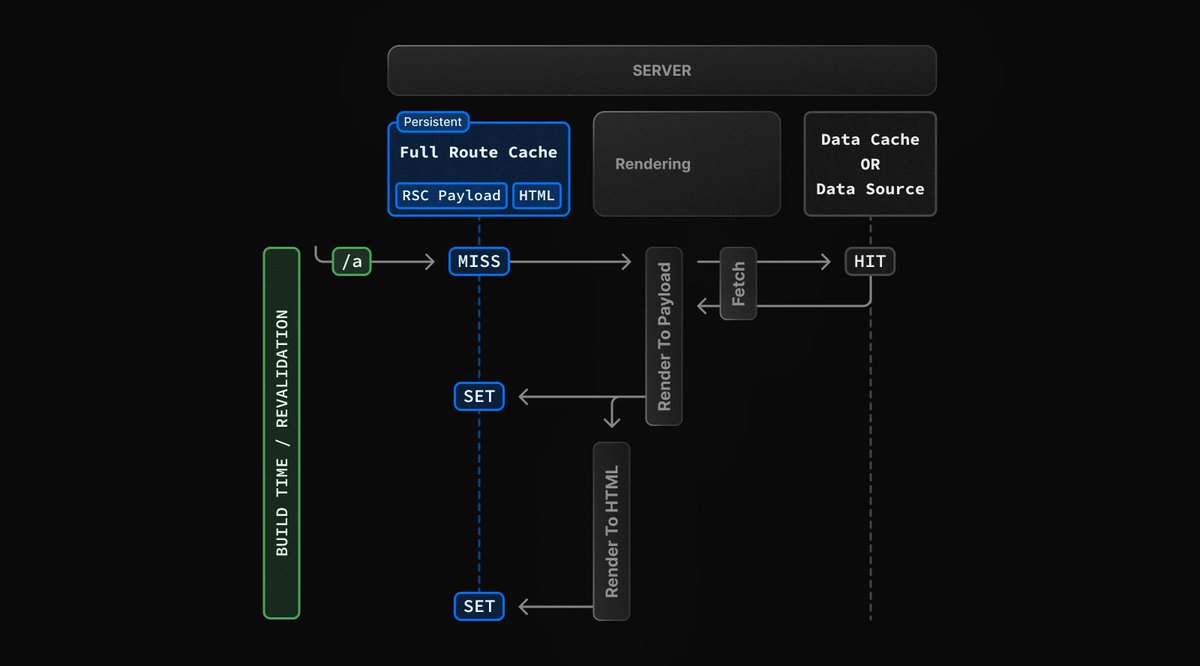
Static vs. Dynamic Rendering
Caching the result of the rendering work is called Static Rendering.
Opting out of caching by using APIs that rely on request-time information and rendering a route on every request is called Dynamic Rendering.
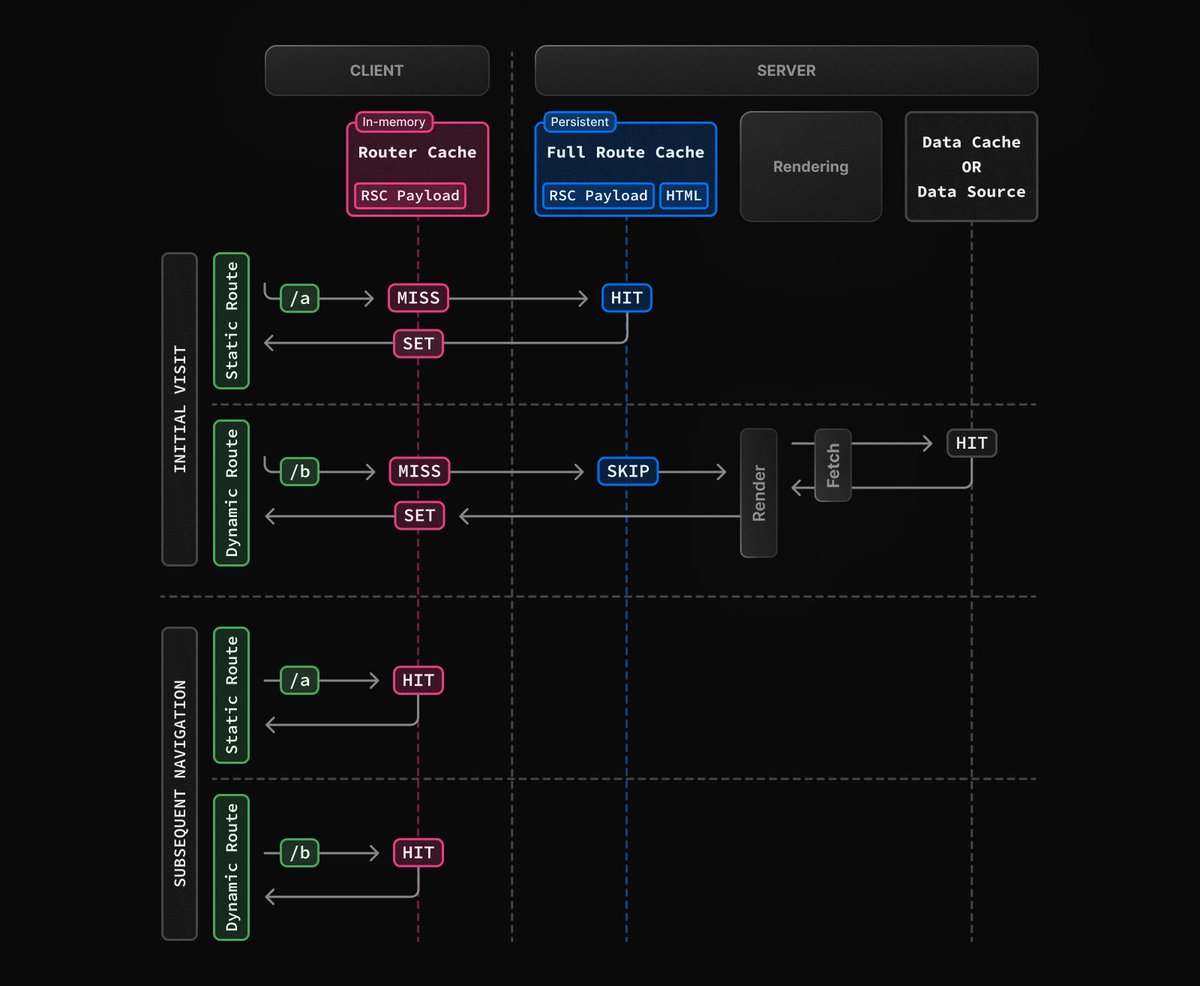
But hold up, because you need to understand something.
In the Pages Router, caching data and caching the rendering result is coupled. E.g. SSG means static rendering w/ cached data, and SSR means dynamic rendering /w uncached data. It's binary, one or the other.
Whereas, in the App Router, rendering is cached by route segment (allows partial/streamable rendering), and data can be cached independently (allows dynamic rendering w/ cached data).
This is why we've avoided using SSG, SSR, ISR, or CSR. The model is different - it's hybrid 😉
📦 Request Memoization
While rendering on the server, React stores the result of fetch requests in a temporary, in-memory cache.
This allows multiple components in a route to share the same data without executing multiple network requests to the data layer.
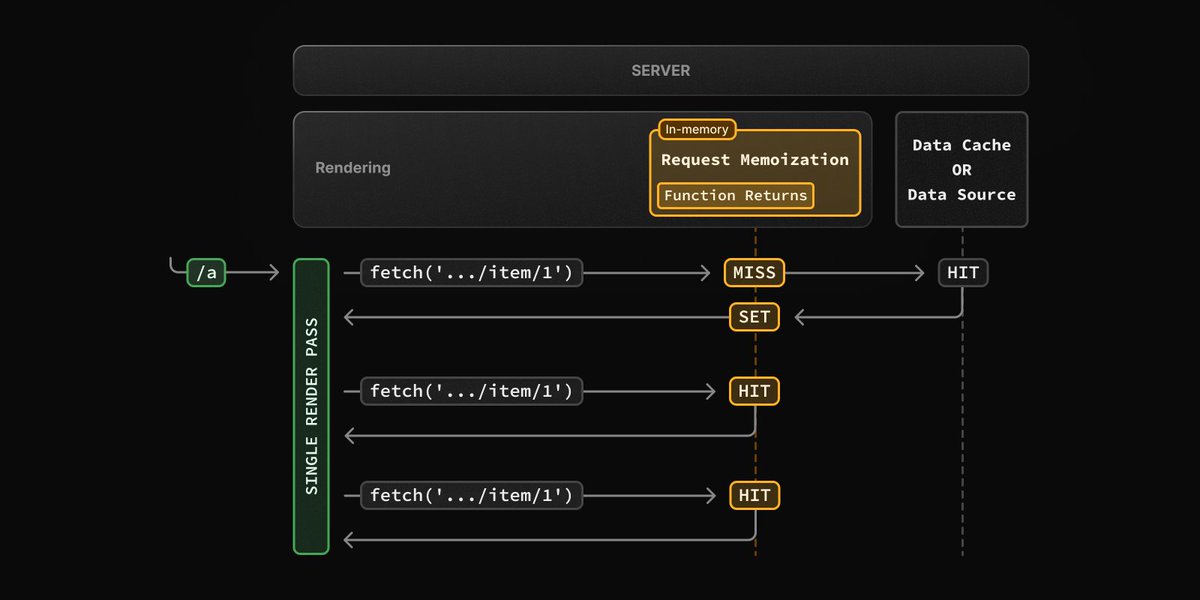
Request memoization allows for a more composable model:
No global data fetching functions, context, or props drilling
- Fetch data where you need it
- Move/delete components without breaking other parts that rely on the same data
- Install packages that fetch their own data
📦 Data Cache
While fetching data on the server, Next.js caches the result in a persistent server-side cache.
This allows cached data to be re-used across server requests and deployments. Significantly improving the performance of dynamic rendering, as some data can be reused.

♻️ Revalidation
Next.js supports fine-grained (by cache tag or route path) revalidation of data, triggered periodically or on-demand based on an event such as a webhook or form submission.
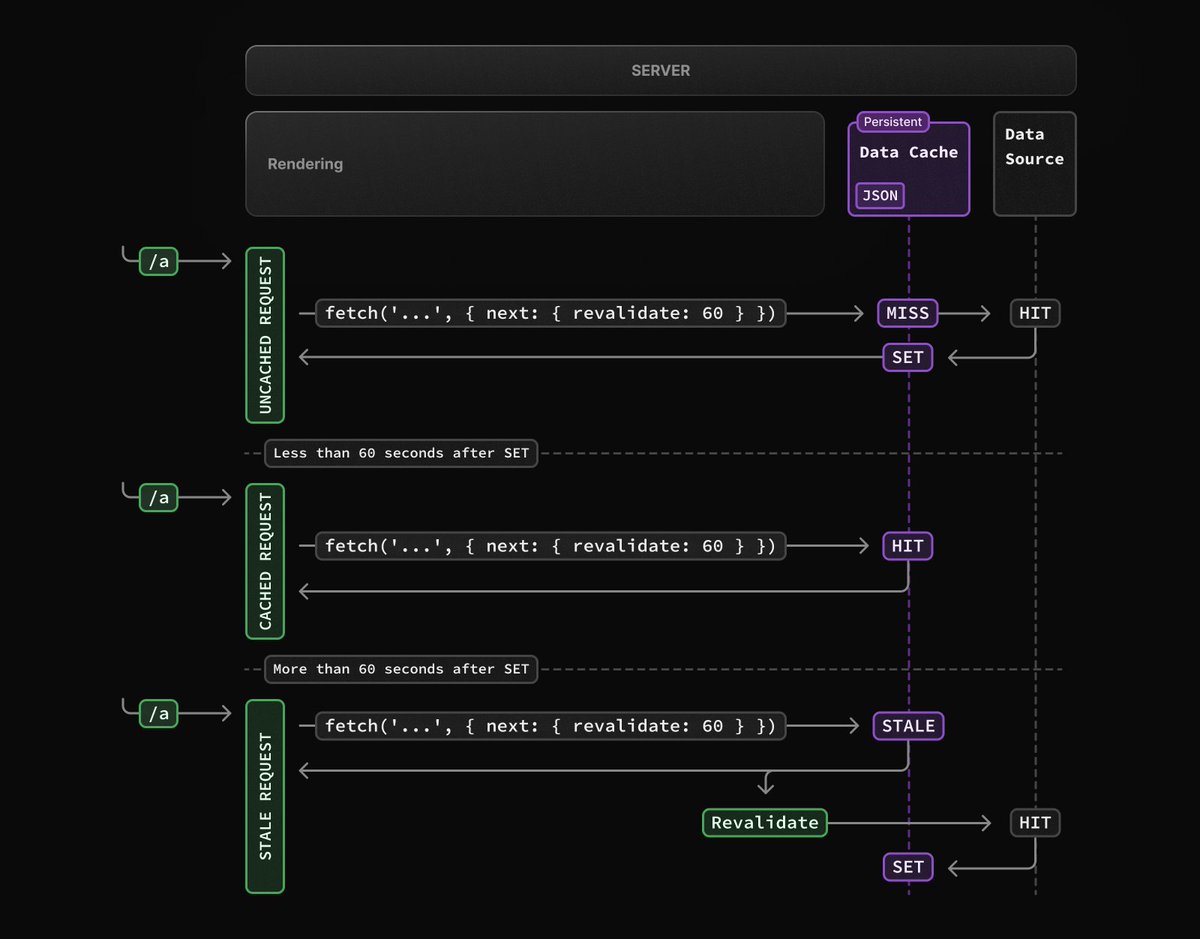

Finally, we're entering a world where you don't have to spend time figuring out what rendering strategy to use.
You decide how to manage your data, and the framework will pick the best rendering strategy and caching semantics based on the APIs you use.